Recordings
Hyperbrowser Session Recordings
Hyperbrowser allows you to record and replay your browser sessions. It uses rrweb, an open-source web session replay library. Session recordings let you:
Visually debug test failures and errors
Analyze user behavior and interactions
Share reproducible bug reports
Save and archive session data
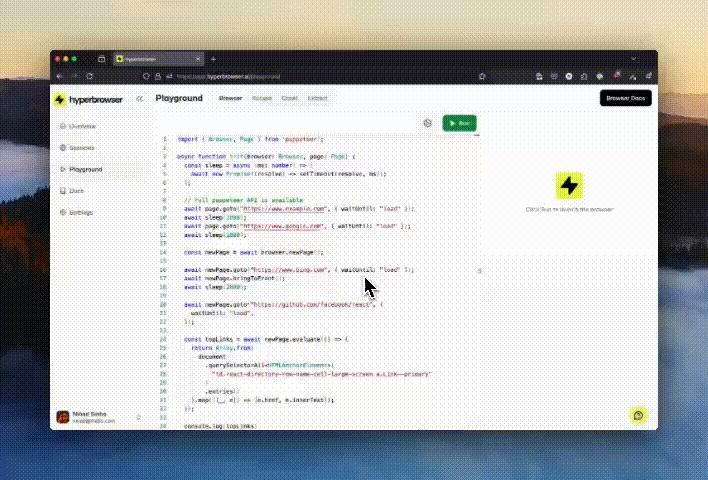
Enabling Session Recording
To record a session, set the enableWebRecording
option to true
when creating a new Hyperbrowser session:
import { Hyperbrowser } from "@hyperbrowser/sdk";
import { config } from "dotenv";
config();
const client = new Hyperbrowser({
apiKey: process.env.HYPERBROWSER_API_KEY,
});
const main = async () => {
const session = await client.sessions.create({
enableWebRecording: true,
});
};
main();
This will record all browser interactions, DOM changes, and network requests for the duration of the session.
Enabling Video Recording
To do a video screen recording which will record the session as a video (.mp4), set the enableWebRecording
and enableVideoWebRecording
options to true
when creating a new Hyperbrowser session:
import { Hyperbrowser } from "@hyperbrowser/sdk";
import { config } from "dotenv";
config();
const client = new Hyperbrowser({
apiKey: process.env.HYPERBROWSER_API_KEY,
});
const main = async () => {
const session = await client.sessions.create({
enableWebRecording: true,
enableVideoWebRecording: true,
});
};
main();
This will capture the screen recording of the session as a video.
Retrieving Recordings
Note the
id
of the session you want to get the recording of.Ensure that the session has been stopped before trying to fetch the recording for it.
Use the Sessions Recording API to get the recordingUrl, or if you are using the SDKs, you can just call the
getRecordingURL
or thegetVideoRecordingURL
function:Poll until the status is either
completed
orfailed
import { Hyperbrowser } from "@hyperbrowser/sdk";
import { config } from "dotenv";
config();
const client = new Hyperbrowser({
apiKey: process.env.HYPERBROWSER_API_KEY,
});
const main = async () => {
const recordingData = await client.sessions.getRecordingURL(
"91e96d43-0dd2-4882-8d3a-613b12583ba2"
);
// or
const recordingData = await client.sessions.getVideoRecordingURL(
"91e96d43-0dd2-4882-8d3a-613b12583ba2"
);
};
main();
The response will have the following data (will be Snake case if using python SDK):
status: "not_enabled" | "pending" | "in_progress" | "completed" | "failed"
recordingUrl: string | null
error: string | null | undefined
If the status
is completed and the recordingUrl
field exists, you can fetch the recording data from that url (via fetch, requests, axios, etc.). The recording data from the url will be returned in rrweb's JSON format for the regular recording and a .mp4 video file for the video web recording.
Replaying rrweb Recordings
Using rrweb's Player
Here's an example of using rrweb's player to replay a recording:
Include the rrweb player script on your page:
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/rrweb@latest/dist/rrweb.min.css"/>
<script src="https://cdn.jsdelivr.net/npm/rrweb@latest/dist/rrweb.min.js"></script>
Add a container element for your player
<div id="player"></div>
Initialize the player with your recording data
// if using rrweb npm package
import rrwebPlayer from "rrweb-player";
import "rrweb-player/dist/style.css";
const recordingData = YOUR_RECORDING_DATA
const replayer = new rrwebPlayer({
target: document.getElementById('player'),
props: {
events: recordingData,
showController: true,
autoPlay: true,
},
});
This will launch an interactive player UI that allows you to play, pause, rewind, and inspect the recorded session.
Building a custom player
You can also use rrweb's APIs to build your own playback UI. Refer to the rrweb documentation for thorough details on how to customize the Replayer to your needs.
Storage and Retention
Session recordings are stored securely in Hyperbrowser's cloud infrastructure. Recordings are retained according to your plan's data retention policy.
Limitations
Session recordings capture only the visual state of the page. They do not include server-side logs, database changes, or other non-DOM modifications.
Recordings may not perfectly reproduce complex WebGL or canvas-based animations.
Last updated